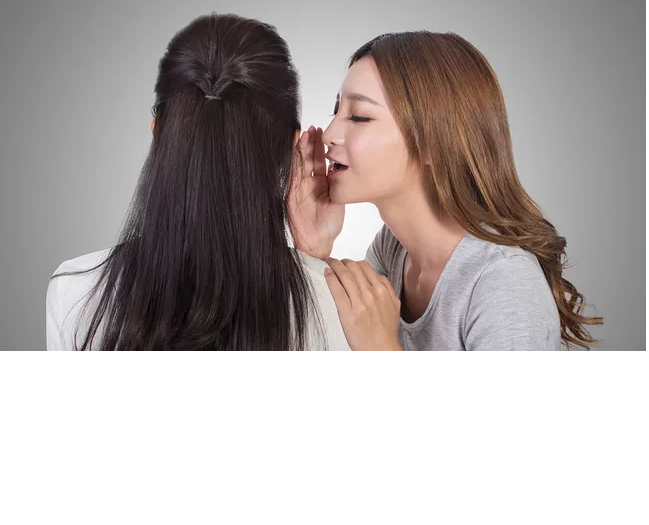
1. preface
Fast Hand released "Seeing" the day before yesterday, received rave reviews, overshadowed the previous "Hou Lang". Nowadays, even content creation has to start playing value-oriented. But the Internet is really a magical thing. We can see what you want without leaving home. Whether it is the current hot chatter, fast hands, or Weixin Official Accounts, Zhihu. You only need to focus on and subscribe to the areas you like, and you can get the content you want and even interact with creators. Creators only need to publish the content they create to the corresponding platform, and users only need to subscribe to their favorite fields or authors on the corresponding platform. Users and creators don't know each other, but they can "see" it. In terms of programming paradigm, this isa publish-subscribe。
2. a publish-subscribe
For example, I am a creator myself, and sometimes I write somethingJava, I'll just callJavais posted; sometimes something else is written, such asPythonSomething like that, hitPythonSend out the label. Readers only need to subscribe to the theme of the corresponding platform (Topic) You can receive push from the article.
The above figure is a simple schematic diagram of publishing and subscribing. Publishing and subscribing consists of the following roles:
- Publisher Publisher. The creator of news is also the source of publishing and subscriptions.
- Msg Message body. It not only contains basic information of the message, but also contains some beacons of the message destination (Topic)。
- Topic theme. Used to establish a pointing relationship between messages and subscribers.
- Broker Also called in many places Channel orEventBus 。When the news comes fromPublisherAfter issuance,BrokerDirect forwarding of messages to the subject (Topic) while maintaining the theme (Topic) Relationship with subscribers.BrokerPublishers and subscribers are completely decoupled.
- Subcriber The ultimate consumer. Consumers subscribe from topics (Topic), the way to obtain the message may beBrokerpush orSubcriberPull.
Advantages of the subscription publishing model: Subscription publishing is event-driven, responsive, and can achieve backpressure and asynchronous. Publishers and subscribers are completely decoupled. You can easily introduce new publishers and new subscribers without having to modify the original code. It is also more suitable for distributed systems.
Of course, it also has drawbacks: first, subscribers may need to poll or iterate to obtain messages. Since publishers and subscribers are completely decoupled, the publisher's publishing status cannot be directly obtained by subscribers, and the subscriber's consumption status cannot be directly obtained by publishers. Secondly, the communication between the two parties is based on the same agreement and requires an agent to complete it. Message specifications and related rules will add some complexity to the system.
3. simple implementation
Here, we simply use Java to implement the above article we write and post it to various UGC platforms, and then the platform pushes it to subscribed fans.
Let's first define an event publishing interface:
package cn.felord;
/**
* Publish interface.
*
* @param <E> the type parameter
* @author felord.cn
* @since 10 :21
*/
public interface Publisher<E> {
/**
* Post events to a topic.
*
* @param topic the topic
* @param event the event
*/
void publish(String topic, E event);
}
Event subscription interface:
package cn.felord;
/**
* Subscription interface.
*
* @author a
* @since 10 :37
*/
public interface Subscriber<E> {
/**
* On event.
*
* @param event the event
*/
void onEvent(E event);
}
thenBrokerAgent, maintenance requiredTopicandSubcriber, push broadcasts on specific topics must also be carried out.This is more suitable for the single-case model:
package cn.felord;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
/**
* The interface Broker.
*
* @author felord.cn
* @since 10 :28
*/
public class Broker {
private final Map<String, Set<Subscriber<String>>> subscribers = new HashMap<>();
private Broker() {
}
private static class Instance {
private static final Broker INSTANCE = new Broker();
}
/**
* 获取单例.
*
* @return the instance
*/
public static Broker getInstance() {
return Instance.INSTANCE;
}
/**
* 对主题进行订阅.
*
* @param topic the topic
* @param subscriber the subscriber
* @return the boolean
*/
public boolean addSub(String topic, Subscriber<String> subscriber) {
if (subscribers.get(topic) == null) {
Set<Subscriber<String>> objects = new HashSet<>();
objects.add(subscriber);
subscribers.put(topic, objects);
}
return subscribers.get(topic).add(subscriber);
}
/**
* 取消订阅.
*
* @param topic the topic
* @param subscriber the subscriber
* @return the boolean
*/
public boolean removeSub(String topic, Subscriber<String> subscriber) {
if (subscribers.get(topic) == null) {
return true;
}
return subscribers.get(topic).remove(subscriber);
}
/**
* 迭代推送事件给订阅者.
*
* @param topic the topic
* @param event the event
*/
public void broadcasting(String topic, String event) {
subscribers.getOrDefault(topic,new HashSet<>()).forEach(subscriber -> subscriber.onEvent(event));
}
}
Next is the publisher's implementation:
package cn.felord;
/**
* @author felord.cn
*/
public class FelordPublisher implements Publisher<String> {
@Override
public void publish(String topic, String event) {
System.out.println("Code farmer Xiaopang is here" + topic + "released one in" + event + "The incident");
Broker.getInstance().broadcasting(topic, event);
}
}
Subscriber implementation:
package cn.felord;
import java.util.Objects;
/**
* 因为需要在Set 中 所以这里最好进行equals hash 覆写
*
* @author felord.cn
*/
public class SomeSubscriber implements Subscriber<String> {
private final String name;
public SomeSubscriber(String name) {
this.name = name;
}
@Override
public void onEvent(String event) {
System.out.println("粉丝 "+name+"接收到了事件 " + event);
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SomeSubscriber that = (SomeSubscriber) o;
return Objects.equals(name, that.name);
}
@Override
public int hashCode() {
return Objects.hash(name);
}
}
Then we can write a short story:
public static void main(String[] args) {
FelordPublisher felordPublisher = new FelordPublisher();
//Published an article
felordPublisher.publish("Java", "Discussion on publish and subscribe models");
//However, there are no subscribers
System.out.println("However, there are no subscribers");
System.out.println("Zhang San subscribed to Java, Li Si subscribed to Python");
Broker.getInstance().addSub("Java", new SomeSubscriber("Zhang San"));
Broker.getInstance().addSub("Python", new SomeSubscriber("Li Si"));
felordPublisher.publish("Java", "Is Java really difficult to learn?");
System.out.println("Wang Wu subscribed to Java");
Broker.getInstance().addSub("Java", new SomeSubscriber("Wang Wu"));
felordPublisher.publish("Java", "For fresh information, visit felord.cn");
//Published Python article
felordPublisher.publish("Python", "Getting started with Python in one day");
}
Print results:
Code farmer Xiaopang is here Java An event related to the publish and subscribe model was published in
However, there are no subscribers
Zhang San subscribed Java, Li Si subscribedPython
Code farmer Xiaopang is here Java A release was published in Java Are events really difficult to learn?
Fan Zhang San received the incident Java Is it really difficult to learn?
Wang Wu subscribedJava
Code farmer Xiaopang is here Java A fresh piece of information was published infelord.cnevents
Fan Zhang San received fresh news about the event and can be accessedfelord.cn
Fan Wang Wu received fresh news about the event and can be accessedfelord.cn
Code farmer Xiaopang is here Python A release was published in Python A one-day entry event
Fan Li Si received the incident Python Getting started in one day
In actual use, attention should be paid toBrokerConcurrent security issues.
5. summary
Today we introduced some related knowledge about subscription publishing, and some of the message middleware we usually useKafka、RabbitMQThese are all manifestations of subscription publishing, and there are also related ideas in the latest responsive programming. So it is still necessary to take a look. There are many articles saying that the observer model and subscription publishing are the same thing; others say that they are not the same thing; there are different opinions and I don't know what you think, so please pay attention to the comments.