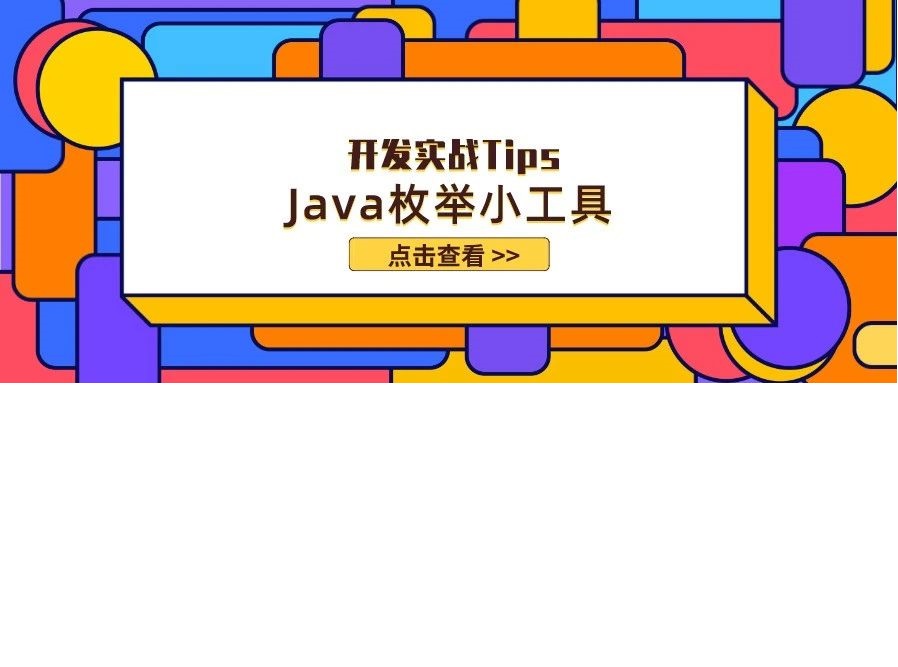
1. preface
JavaEnumerations are very useful in development. Today, let's analyze a few tips and answer some students 'questions. The first thing to explain is that my enumeration is based on the following paradigm:
2. How to bind enumeration values to drop-down list
This scenario is very common. If you encapsulate attributes such as states and categories into an enumeration structure, like below, one identifier corresponds to one state, which is a typical drop-down list structure.
public enum EnabledEnum implements Enumerator {
/**
* Disable status enum.
*/
DISABLE(0, "Not available"),
/**
* Enable status enum.
*/
ENABLE(1, "Available");
private final int value;
private final String description;
EnabledEnum(int value, String description) {
this.value = value;
this.description = description;
}
@Override
public int value() {
return this.value;
}
@Override
public String description() {
return this.description;
}
}
The front-end wants to be able to obtain these states to fill in the drop-down list. How do you parse and encapsulate it? I have two ways here. The first one you can start from JSON Class Library Jackson Elegantly Serializes Java Enumeration ClassesGet the solution in one article; the second one is simpler, just write a tool class.
public static <E extends Enum<?& gt; & Enumerator> Map<Integer, String> enumToMap(Class<E> clazz) {
E[] enumConstants = clazz.getEnumConstants();
Map<Integer, Object> map = new LinkedHashMap<>();
for (E e : enumConstants) {
map.put(e.value(), e.description());
}
return map;
}
use anJava 8new features additional constraint。that isextends
You can go through later&
Symbol has additional constraints and can be reused. Note that it must be an interface type, not an abstract class or otherClass
。Represents that the upper bound of generics is subject to multiple constraints.<E extends Enum<?& gt; & Enumerator>
can be interpreted asE
Must be an enumeration class and must also implement theEnumerator
Interface.
Why achieve
Enumerator
Interface? The first advantage is that a unified paradigm can be defined; the second advantage is that there is no need to transform when analyzing it.
I tried usingStream
I came to encapsulate but found it too complicated. Although it was successful, it was a bit difficult to understand. I also share it here:
public static <E extends Enum<?& gt; & Enumerator> Map<Integer, String> enumToOptions(Class<E> enumClazz){
//Check whether the key is duplicate during merge
BinaryOperator<String> merge = (u, v) -> {
throw new IllegalStateException(String.format("Duplicate key %s", u));
};
Enumerator[] enumConstants = enumClazz.getEnumConstants();
return Stream.of(enumConstants)
.collect(Collectors.toMap(Enumerator::value,
Enumerator::description,
merge,LinkedHashMap::new));
}
3. How to find enumerations based on values
This is also very common, and the most direct way is to write a switch Statement. But writing one for each class is very cumbersome. So you can also write a tool class for this, and use this timeStream
It's much simpler.
public static <E extends Enum<?& gt; & Enumerator> Optional<E> getEnumByValue(Class<E> enumClazz, final Integer value){
return Stream.of(enumClazz.getEnumConstants())
.filter(enumerator -> Objects.equals(enumerator.code(),value))
.findAny();
}
return
Optional
Because it's possible to givevalue
There is no corresponding enumeration.
4. summary
Today, I shared two gadget classes to operate enumeration. Not only do I use some knowledge of enumeration, but I also useJava 8Three new features:Generic additional constraints、Optional