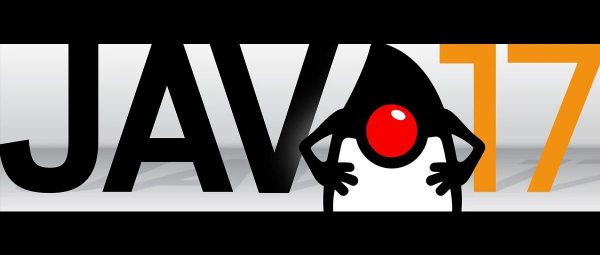
Java 17 is one of the most important LTS versions of Java, but the direct transition from Java 8 to Java 17 is too long and is bound to be difficult. So Fat Brother took the time to sort out the changes in some commonly used APIs from Java 9 to Java 17. Today, let's take a look at what Java 9 has.
Java 9
The biggest change in Java 9 is the introduction of a JShell and modularity. It doesn't use it much every day, so I don't spend time on these functions today.
New way to create collections
Used GoogleGuavaClass libraries know,GuavaProvides a static factory method for creating collections and the ability to infer generics. For example:
List<Person> list = Lists.newArrayList();
Map<KeyType, Person> map = Maps.newLinkedHashMap();
And the original ecology requires all kinds ofnew
to define. This has been improved in Java 9, and now you can:
// [1, 2, 3, 4]
List<Integer> integers = List.of(1, 2, 3, 4);
// {1,2,3}
Set<Integer> integerSet = Set.of(1, 2, 3);
// {"hello":"world","hi":"java"}
Map<String, String> hello = Map.of("hello", "world", "hi", "java");
But please note: The collections created by these APIs are Immutable, and you cannot add or delete these collections.
Stream extension
The Stream API is one of the most important features introduced in Java 8. in Java 9Stream
It has been further strengthened.
ofNullable
Stream<T> ofNullable(T t)
Returns the order containing individual elementsStream
, if not empty, otherwise return emptyStream
。This is relatively simple, so I won't give you an example.
iterate
Stream<T> iterate(T seed, Predicate<? super T> hasNext, UnaryOperator<T> next)
This is a new iterative implementation used to generate finite flows.
seed
initial seed valuehasNext
Used to determine when to end the flow, this is related toseed
related. How to keep this function without iterationseed
Calculated, the returned stream may be empty.next
Function is used to calculate the value of the next element.
An example:
Stream.iterate(0, i -> i < 5, i -> i + 1)
.forEach(System.out::println);
Equivalent to traditional:
for (int i = 0; i < 5; ++i) {
System.out.println(i);
}
takeWhile
Stream.takeWhile(Predicate)
Stream
Elements in will be assertedPredicate
, once the element is asserted asfalse
The operation is interrupted, elements that are not asserted are ignored (if any of the elements in the unasserted meet the conditions), and only the previously satisfied elements are returned.
Stream.of(1, 2, 3, 4, 2, 5)
.takeWhile(x -> x < 4)
.forEach(System.out::println);
In the above example, only output1
、2
、3
。
dropWhile
This API andtakeWhile
The mechanism is similar and is also used to screenStream
Elements in. However, elements that match the assertion will be retrieved fromStream
Remove from. Once an element is asserted asfalse
, will assert thatfalse
and all subsequent elements are returned.
Stream.of(1, 2, 3, 4, 2, 5)
.dropWhile(x -> x < 4)
.forEach(System.out::println);
The example above will output4
、2
、5
。
and
filter
The operation is different, remember!
Optional extension
Optional
Three useful APIs have been added.
stream()
Optional
You can transfer nowStream
。ifPresentOrElse(Consumer<? super T> action, Runnable emptyAction)
How to consume if it is worth it, and how to consume if it is not worth it.or(Supplier<? extends Optional<? extends T>> supplier)
If there is a value, return the valueOptional
, otherwise it provides a method that can obtain a valuableOptional
channel (Supplier
)。
try-with-resources optimization
Introduced in Java 7try-with-resourcesFunction that ensures that each declared resource will be closed at the end of the statement. Any realizationjava.lang.AutoCloseable
Interface, and implements thejava.io.Closeable
Interface objects can be used as resources.
You need to write this in Java 7:
try (BufferedInputStream bufferedInputStream = new BufferedInputStream(System.in);
BufferedInputStream bufferedInputStream1 = new BufferedInputStream(System.in)) {
// do something
} catch (IOException e) {
e.printStackTrace();
}
Java 9 simplified it to:
BufferedInputStream bufferedInputStream = new BufferedInputStream(System.in);
BufferedInputStream bufferedInputStream1 = new BufferedInputStream(System.in);
try (bufferedInputStream;
bufferedInputStream1) {
// do something
} catch (IOException e) {
e.printStackTrace();
}
Interface private method
Following the introduction of interface static methods and interface default methods in Java 8, it introduced interface private methods:
public interface Catable {
/**
* Interface private method
*/
private void doSomething() {
}
}
Introducing HttpClient
Define a new HTTP client API to implement HTTP/2 and WebSocket and replace the oldHttpURLConnection
API。Java used to be native to it, so easy-to-use clients such as Apache HttpClientComponents and OkHttp were born. The new one doesn't work very well, but it's still from zero to one.
HttpRequest httpRequest = HttpRequest.newBuilder(newURI)
.header("Content-Type","*/*")
.GET()
.build();
HttpClient httpClient = HttpClient.newBuilder()
.connectTimeout(Duration.of(10, ChronoUnit.SECONDS))
.version(HttpClient.Version.HTTP_2)
.build();
Flow
Spring WebFluxThe responsive Web framework has been around for four years, and the reactive streams specification has also been initially introduced into the JDK in Java 9. This thing is still a bit advanced at present. Brother Fat hasn't found a specific application scenario yet, so I'll dig a hole first.
summary
In fact, Java 9 still has some underlying optimizations, but it is enough for ordinary developers to understand these. Among the above features, the more commonly used ones are static invariant sets,try-with-resourcesOptimize. Other features will only add to the cake if you are very familiar with Java 8.
Comments0