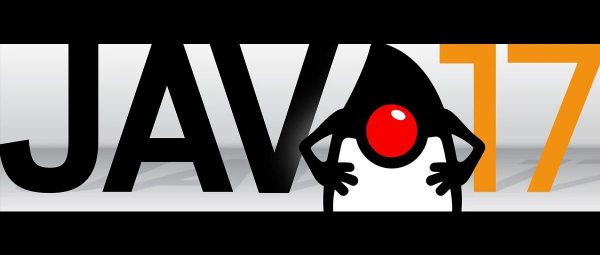
There are not many features that are useful to developers in Java 12, but it is still useful.
String enhancement
Java 12 further enhances string operations, adding two methods.
String indent
String.indent(int n)
will be based on parametersn
Indent the string. The specific rules are
- when
n>0
is inserted at the beginning of each line of the stringn
Space, and the entire string moves right. - when
n<0
is deleted at the beginning of each line of the stringn
spaces, if the actual number of spaces is less thann
, deletes all spaces on the line, but does not wrap the line.
Let's experiment:
String text = " Hello \n Java12";
System.out.println("Before indenting");
System.out.println(text);
System.out.println("Indent two characters to the right");
String indent2 = text.indent(2);
System.out.println(indent2);
System.out.println("Three characters are indented on the left side, but there is actually only one space");
String indent3 = text.indent(-3);
System.out.println(indent3);
The corresponding result is:
string conversion
String
added atransform
Method to functionalize string operations.
<R> R transform(Function<? super String, ? extends R> f)
The purpose is to strengthen the functional operation of strings. An example:
String txt = "hello ";
// hello hello
String s = txt.transform(str -> str.repeat(2));
Every version of Java strengthens functional programming.
Content-based file matching
Java 12 inFiles
A new static method has been added to the tool classFiles.mismatch(Path,Path)
, used to find the content of two documents (byte
) If there is a difference, return the position of the first mismatch byte in the contents of the two files. If there is no mismatch, return-1L
。
//File comparison
Path p1 = Files.createTempFile("file1", "txt");
Path p2 = Files.createTempFile("file2", "txt");
Files.writeString(p1, "felord.cn");
Files.writeString(p2, "felord.cn");
// -1L The content is the same
long mismatch = Files.mismatch(p1, p2);
This method and another methodFiles.isSameFile(Path, Path)
The effects are somewhat similar, but there are still differences.
Collectors::teeing
Aggregation operations on Stream streamsCollector
Further enhanced and increasedteeing
Operations to implement some complex aggregation operations. For example, if I want to count the proportion of the average of an array in the total, I have to first calculate the average, then calculate the total, and then divide. This requires three steps.
Double average = Stream.of(1, 2, 3, 4, 5).collect(Collectors.averagingDouble(i -> i));
Double total = Stream.of(1, 2, 3, 4, 5).collect(Collectors.summingDouble(i -> i));
Double percentage = average / total;
usedteeing
You can then complete it in one step:
Double meanPercentage = Stream.of(1, 2, 3, 4, 5)
.collect(Collectors.teeing(
Collectors.averagingDouble(i -> i),
Collectors.summingDouble(i -> i),
(average, total) -> average / total));
New digital formatting
Java 12 introduces new region-based compact digital format classesCompactNumberFormat
, used to simplify long numbers. Usually programmers like to label the salary range as10k-20k
, while some other industries like10000-20000
。
NumberFormat chnFormat = NumberFormat.getCompactNumberInstance(Locale.CHINA,
NumberFormat.Style.SHORT);
chnFormat.setMaximumFractionDigits(3);
//82.32 million
String cformat = chnFormat.format(82323);
NumberFormat usFormat = NumberFormat.getCompactNumberInstance(Locale.US,
NumberFormat.Style.SHORT);
usFormat.setMaximumFractionDigits(3);
// 82.323K
String uformat = usFormat.format(82323);
You can also customize
CompactNumberFormat
to achieve personalized digital formatting.
other
In addition to the above, Java12 also has some preview properties and JVM enhancements, but there are not many highlights.
Comments0