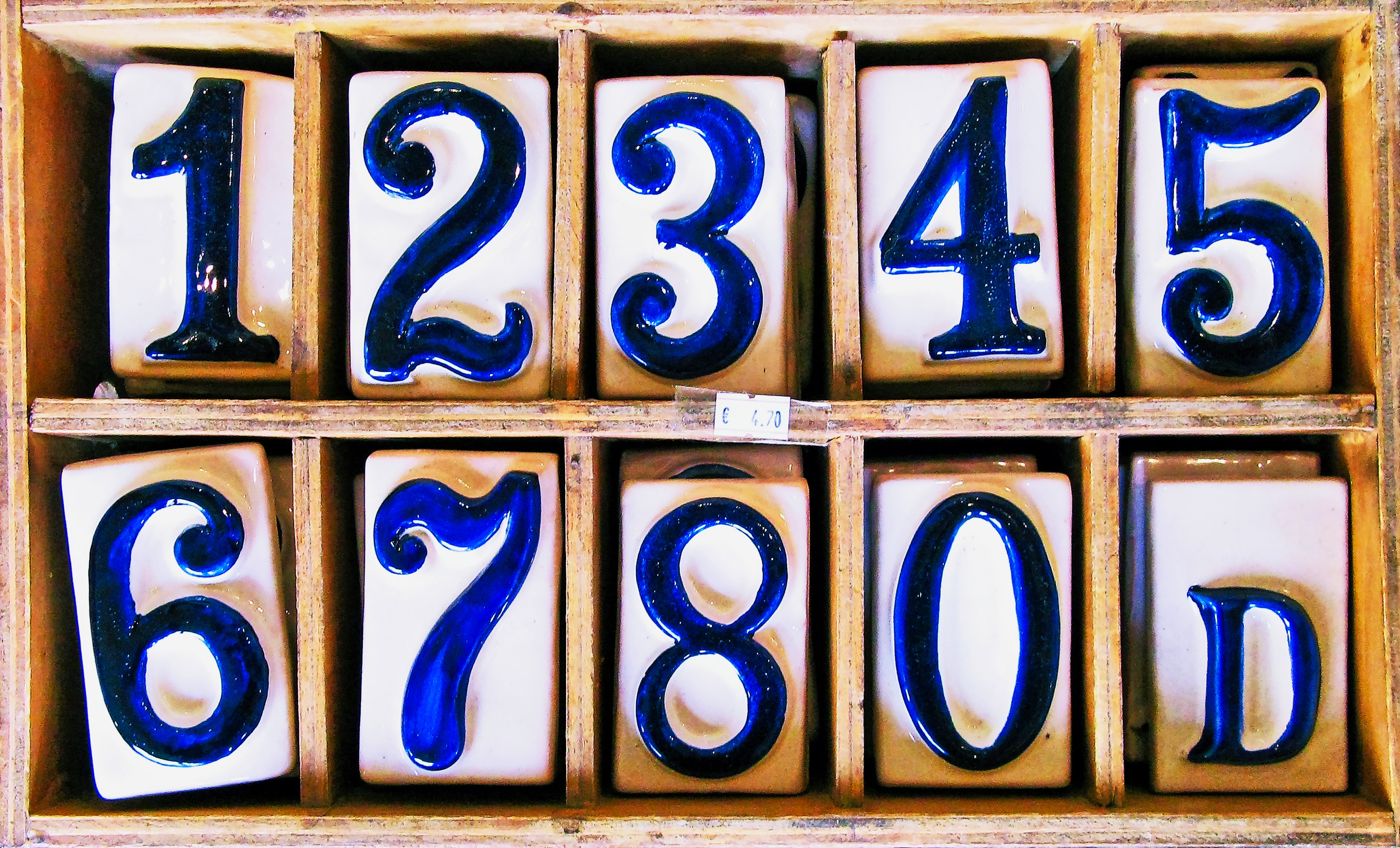
preface
Numbers are often represented and conveyed in strings in some fields. So how do we determine whether a string is a number? Today we will discuss this topic.
Empty characters and null
First of all, we can clearly know the empty characters""
andnull
Definitely not numbers. In fact, we write other logic the same. Give priority to some of the most extreme and easily identifiable logical judgments. This is a small tip.
toCharArray
Strings that exclude previous situations can be passed throughtoCharArray()
Method to convert to a char array. andCharacter.isDigit(int)
It's easy to determine whether the char element is a number (don't ask why char is an int!). So will this method work? Let's operate a wave of various situations:
public class Main {
public static void main(String[] args) {
// false
System.out.println("\"\" 是不是数字: "+isNumeric(""));
// false
System.out.println("\" \" 是不是数字: "+isNumeric(" "));
// false
System.out.println("null 是不是数字: "+isNumeric(null));
// false
System.out.println("1,200 是不是数字: "+isNumeric("1,200"));
// true
System.out.println("1 是不是数字: "+isNumeric("1"));
// 预期是负数 却为 false
System.out.println("-1 是不是数字: "+isNumeric("-1"));
// true
System.out.println("200 是不是数字: "+isNumeric("200"));
// 预期是保留两位的浮点数 却为false
System.out.println("3000.00 是不是数字: "+isNumeric("3000.00"));
// 二进制
System.out.println("0b11001 是不是数字: "+isNumeric("0b11001"));
// 八进制 true
System.out.println("012 是不是数字: "+isNumeric("012"));
// 十六进制 false
System.out.println("0x12 是不是数字: "+isNumeric("0x12"));
// A-F 代表十六进制中的 10-15 false
System.out.println("0xAF 是不是数字: "+isNumeric("0xAF"));
// double false
System.out.println("12.12d 是不是数字: "+isNumeric("12.12d"));
// double 科学计数法 false
System.out.println("12E4 是不是数字: "+isNumeric("12E4"));
// float false
System.out.println("12.123f 是不是数字: "+isNumeric("12.123f"));
// 分隔符 jdk1.7 false
System.out.println("1_000_000 是不是数字: "+isNumeric("1_000_000"));
}
public static boolean isNumeric(final String str) {
// null or empty
if (str == null || str.length() == 0) {
return false;
}
return str.chars().allMatch(Character::isDigit);
}
}
As can be seen from the above, there are not many problems with decimal positive integers that are moderate to moderate. Once it is floating point numbers, decimal numbers, negative numbers, binary, hexadecimal, scientific notation, and separators, this method becomes less easy to use. It suddenly occurred to me that there were some methods available in packaging.
parse
Numbers have corresponding packaging categoriesparse
method. Throw if the string does not meet the rules for the corresponding number typeNumberFormatException
abnormal. So let's change our judgment here:
public static boolean isNumeric(final String str) {
// null or empty
if (str == null || str.length() == 0) {
return false;
}
try {
Integer.parseInt(str);
return true;
} catch (NumberFormatException e) {
try {
Double.parseDouble(str);
return true;
} catch (NumberFormatException ex) {
try {
Float.parseFloat(str);
return true;
} catch (NumberFormatException exx) {
return false;
}
}
}
}
Implement it again, and the following results are obtained:
"" Is it a number: false
" " Is it a number: false
null Is it a number: false
1,200 Is it a number: false
1 Is it a number: true
-1 Is it a number: true
200 Is it a number: true
3000.00 Is it a number: true
0b11001 Is it a number: false
012 Is it a number: true
0x12 Is it a number: false
0xAF Is it a number: false
12.12d Is it a number: true
12E4 Is it a number: true
12.123f Is it a number: true
1_000_000 Is it a number: false
Starting from the fifth line above, the representation of numbers is supported by java. Judging from the execution results, everything except binary, hexadecimal, and separators met expectations. Although this method is not perfect, we can also learn some rules corresponding to the parse method. That's the point.
third-party class libraries
In other words, the api provided by jdk does not have silver bullets. So is there a third-party library to detect it? We used the common lang3 library (version 3.9)NumberUtils
Tool class to process, IisParsable
、isDigits
、isCreatable
Methods were tested separately and found thatisCreatable
The method works best, onlydelimiter
We did not meet our expectations. If you don't consider this situation, it should beisCreatable
Basically enough to meet your needs.
regular expression
In fact, using regular expressions is the most perfect solution to the problem.
summary
Today's verification of whether a string is a number type in java allows us to review how numbers are represented and converted in java. Some rules were discovered. I believe this article will make you have a deeper impression on the numbers in java, and I hope it will be helpful to your work.
Comments0