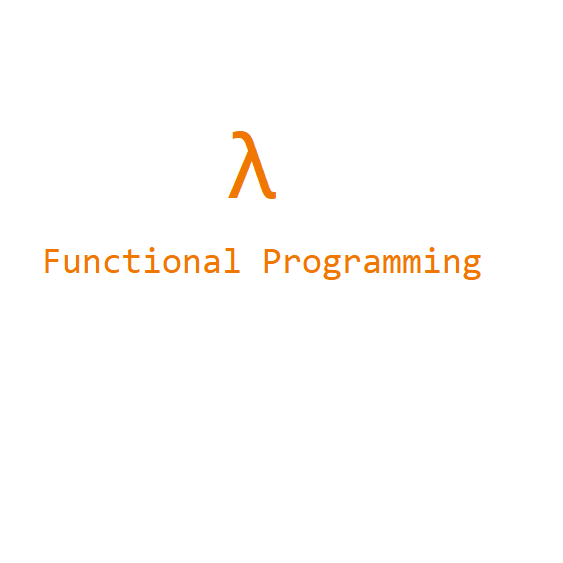
1. preface
I have been busy recently, and I have less and less time to write. I have been experimenting with functional programming styles in Java development these days. So I wrote something to share.
2. What is functional programming?
In my opinion, functional programming means using functions as first-class citizens. Normally, we process data in development. Classes are being processed in object-oriented. Functional programming is either writing functions or on the way to writing functions. Let's take a look at the evolution of functional programming in Java. When I was a kid, I played a cheap black-and-white screen game console and could only play Tetris. This is not scalable.
public void playGame(){
//Only play Tetris
}
This kind of machine brought a lot of fun to childhood. Later, my friend got a gameboy. The advantage of this handheld is that it can be inserted into the card. One card, one game, the most famous ones are "Super Mario" and "Brick Breaking". At this time, the machine is fully scalable.
public void playGameboy(GameboyCard card){
card.getGame().run()
}
Although it could be expanded, the price of the card was very expensive, and there were still few channels to buy cards at that time. At that time, I actually thought it would be great if I could build my own gameboy game (and then I would do programming?). The ideal game console is one where we don't care about your game style, as long as you can put it into a game card that matches the interface and run in my game console.
So we defined a fixed game card interface:
/**
* @author Felordcn
* @since 2019/10/31 22:13
*/
@FunctionalInterface
public interface Card {
Game apply();
}
As long as game cards that match this interface can be plugged into the machine to play:
/**
* Fun.
*
* @param card the card
*/
void fun(Card card) {
Game game = card.apply();
game.run();
}
Students skilled in object-oriented will say, isn't this interface-oriented programming? Yes, there is no problem with what you said. but here Card
The only thing the interface does is provide games. Is our focus on game cards? Obviously not! Fun and fun games are our goal. So whether it is a card, a CD or even the Internet, as long as it can provide us with games for entertainment, it meets our needs.
//Play card game console
fun(() -> new CardGame());
//Play PSP
fun(() -> new PSPGame());
// more
As code farmers, we usually write SQL。Whether it is a large factory or a small factory, whether it is single or distributed.SQL It always helps us resolve many business relationships.SELECT
、 INSERT
、 UPDATE
、 DELETE
Every order is SQL Standardize the database, no matter what table it is, it will operate consistently. You perform whatever orders you declare. In this case, the data and the function are loosely coupled. It is this characteristic that allows us to achieve "every change is consistent with its origin." This is also an alternative form of functional programming.
3. Do object-oriented and functional programming conflict?
Object-orientation has always been in the paradigm of what data I can manipulate and how I should manipulate that data. Functional programming has always been immersed in giving me ways to manipulate data. The biggest advantages of object-orientation are polymorphism and encapsulation; the advantages of functional programming are abstraction and declarative command styles. The two are actually orthogonal, complementary, and can coexist in the same program. Debating whether object-oriented or function-oriented is as extreme as debating which language is better. For object-oriented: not everything that exists is necessarily an object, a function is an object; for functional programming: what exists is not always pure, and side effects are always real. In short, object-oriented focuses on decomposition, and functional programming focuses on composition.
4. Functional Programming Features
Functional style programming has some of its own characteristics:
- Function as a first-class citizen. It can be passed as an argument, returned from a function, or assigned to a variable.
- with closures Lambda Expressions and anonymous functions, which are widely polymorphic.
- Invariant, most stateless treatment. In functional programs, variables are obtained through external pass or declaration. Variables cannot be changed
- Calls based on immutability and thus without side effects.
- by
tail call
Implement recursive performance optimization. - Provide dynamic and composable development ideas.
5. summary
Today I briefly expressed some of my understanding of functional programming. For Java developers who are accustomed to object-oriented, understanding functional programming is not easy. It's not just Lambda And anonymous functions! It's more of an idea. Recommended a good one here Java Functional programming library vavr 。Interested students can learn.