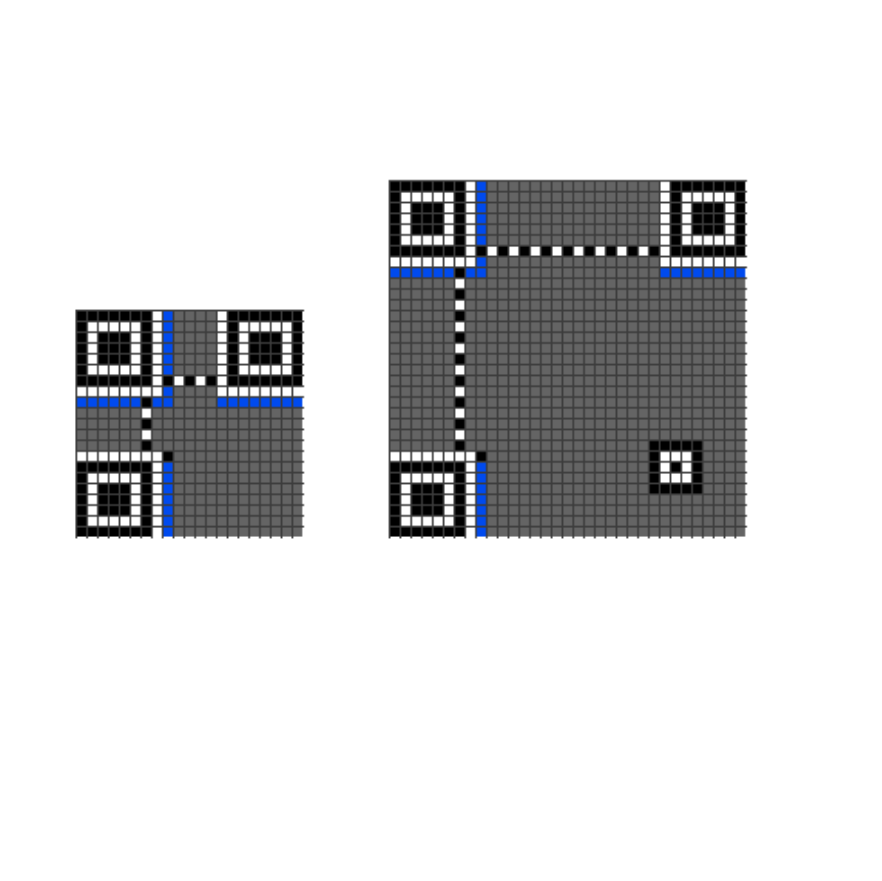
1. preface
I don't know when it started, but our lives were suddenly filled with QR codes. Even the auntie's egg cake breakfast stand on the street was posted with QR codes. Moreover, QR codes were also used for the control of this epidemic to avoid cross-infection caused by manual filling. so Java How to develop QR code functions? Let's have a brief discussion today.
2. About QR code
As developers, we will definitely think that QR codes are patterns that encode content into QR codes. We don't need to know how it works, it's just a process of encoding and decoding. But we still need to know something about QR codes during development:
- Exactly the same QR code has exactly the same content as long as the algorithm is consistent, and the contrary does not hold true.
- The more content the QR code, the more complex it is, which can be seen with the naked eye from the image of the QR code. Which means the more time it takes to decode.
- QR codes have a fault tolerance rate. The higher the fault tolerance rate, the greater the amount of information the QR codes contain.
Based on the above points, we will make some adjustments based on actual conditions during development, and I will talk about my own experience later.
3. Java implements the generation of QR codes
usually we use Google open source 1D/2D Bar code image processing library ZXing to achieve it. We can integrate QR code generation functionality by introducing its dependencies:
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.4.0</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.4.0</version>
</dependency>
Then we can generate a QR code using the following few lines of code and save it locally:
QRCodeWriter qrCodeWriter = new QRCodeWriter();
#The first parameter is the content of the QR code, the second parameter remains unchanged, the third and fourth parameters are width and height in turn
BitMatrix bitMatrix = qrCodeWriter.encode("https://www.felord.cn", BarcodeFormat.QR_CODE, 30, 30);
#Save the QR code as a png local image.
MatrixToImageWriter.writeToPath(encode, "png", Paths.get("E:\\workbase\\qr.png"));
If you want to control the encoded character set and error correction rate, the above code can be changed to:
QRCodeWriter qrCodeWriter = new QRCodeWriter();
Map<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET,"UTF-8");
BitMatrix bitMatrix = qrCodeWriter.encode("https://www.felord.cn", BarcodeFormat.QR_CODE, 80, 80,hints);
#Save the QR code as a png local image.
MatrixToImageWriter.writeToPath(encode, "png", Paths.get("E:\\workbase\\qr.png"));
in fact MatrixToImageWriter
Not only does it provide saving QR codes as files, but it can also be converted into streams:
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
MatrixToImageWriter.writeToStream(bitMatrix,"png",byteArrayOutputStream);
byte[] bytes = byteArrayOutputStream.toByteArray();
String base64Image = new BASE64Encoder().encode(bytes);
generated base64Image
We can display it on the front end in the following ways:
<img src="data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAFAAAABQAQAAAACmas8AAAAAvklEQVR42tXSsRGDMAwFUKWxV0jl
rGY39gpOg6BxVoDGWQ0aewVorOQOzgeCARJVr9G/rzsB1YEfZgHh5QjIiPn1zoVTu6SsOLPIdMEh
2BMxJ1lzK0FYq4GRqJgWt76V5e665Na1HQ1F09/oSIpu6qaIRxaztG5pOZXrmy1sRzPjqJ7EKIQ2
Ye27o8clD4ETJxrB0JHfg7xqOAvIrtgGGTFH3XhObS3ABTHn+UQXXAROTI88a04QGB6R8Q9e+QOH
lnNzjaH0oAAAAABJRU5ErkJggg==" alt="">
Base64 There is no problem displaying a small QR code, if generated Base64 A longer string will cause greater rendering consumption. The pros and cons must be weighed in actual production.
4. dynamic QR code
If we need to generate QR codes dynamically, or in other words, use QR codes as a service. We can use Servlet To implement a dynamic QR code service. we use Spring MVC to achieve:
package cn.felord.qr.format;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.QRCodeWriter;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.ServletOutputStream;
import javax.servlet.http.HttpServletResponse;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
/**
* @author dax
* @since 2020/2/29 21:09
*/
@Controller
@RequestMapping("/qr")
public class QRController {
@GetMapping("/felord")
public void gen(HttpServletResponse response) throws IOException, WriterException {
response.setContentType("image/png");
ServletOutputStream outputStream = response.getOutputStream();
outputStream.write(imageBytes());
outputStream.flush();
outputStream.close();
}
private byte [] imageBytes() throws IOException, WriterException {
QRCodeWriter qrCodeWriter = new QRCodeWriter();
Map<EncodeHintType, Object> hints = new HashMap<>();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.H);
hints.put(EncodeHintType.CHARACTER_SET,"UTF-8");
BitMatrix bitMatrix = qrCodeWriter.encode("https://www.felord.cn", BarcodeFormat.QR_CODE, 80, 80,hints);
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
MatrixToImageWriter.writeToStream(bitMatrix,"png",byteArrayOutputStream);
return byteArrayOutputStream.toByteArray();
}
}
5. Some practical experiences
In actual production, we should pay attention to the following points:
- Try to avoid transmitting sensitive plaintext information in QR codes, and should be summarized or desensitized.
- For relatively long URLs, short URL services should be used to reduce the information load of QR codes.
- Try to ensure the uniqueness of the QR code within a certain period of time, such as adding some meaningless random values.
- In fact, there are other powerful out-of-the-box zxing secondary encapsulation libraries available, such as qrext4j
6. summary
today Java This paper discusses the development of QR codes, from some characteristics of QR codes to ZXing's generation of QR codes and development of them as services. Finally, it lists some key points in actual use, hoping it will be useful to you. Welcome to express your opinions and questions through leave a message.